When trying to run the example python file for CAN bus
import time
import os
import sys
import can
# Using the extension we must setup the can interface within Python
os.system("ip link set can0 type can bitrate 500000")
time.sleep(1)
os.system("ip link set can0 up")
time.sleep(1)
# set up a CAN bus
bus = can.interface.Bus(channel="can0", bustype='socketcan')
# Our Transmission ID
tx_arb_id = 0x123
# Our CAN Frame
obd_req_data = [0xDE, 0xAD, 0xBE, 0xEF]
# Send our message in CAN 11-bit format
msg = can.Message(arbitration_id=tx_arb_id, data=obd_req_data, is_extended_id=False)
bus.send(msg)
message = bus.recv(10.0) # Quite a good timeout for a demonstration!
if message is None:
print('Timeout occurred, no message.')
sys.exit(1)
# Then we print the received message if any
print(str(message))
I get quite a few error messages:
Import "can" could not be resolved Pylance(reportMissingImports) [ln 4 col 8]
Cannot read properties of undefined (reading ‘0’)
and finally a dialogue:
Invalid message: “noDebug” is not suppoorted for “attach”
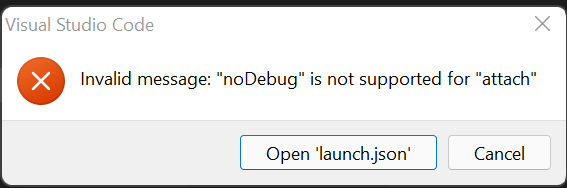
Here is my configuration
my terminal when I click “run python File”:
Windows PowerShell
Copyright (C) Microsoft Corporation. All rights reserved.
Install the latest PowerShell for new features and improvements! Migrating from Windows PowerShell 5.1 to PowerShell 7 - PowerShell | Microsoft Learn
PS C:\GitHub3\CANhandler\CANhandler> & C:/Users/LeighBoyd/AppData/Local/Programs/Python/Python310/python.exe c:/GitHub3/CANhandler/CANhandler/main.py
Traceback (most recent call last):
File “c:\GitHub3\CANhandler\CANhandler\main.py”, line 4, in
import can
ModuleNotFoundError: No module named ‘can’
Questions:
- Should I be running this from a Development container? I notice the python.exe file was located on C:/users/… i.e. in Windows. this can’t be right.
- Should I be doing a “run without debugging” to execute this instead of upper right “Run Python File”
- is there an equivalent C example? I am not sure how to combine my existing C project with Python (or do you think I should run this as a separate container and use a docker network to send over the CAN info to my application?)
Thanks!
Greetings @leighjboyd,
when I click “run python File”:
Wait are you saying you’re running the Python: Run Python File in Terminal
. As in this Visual Studio Code command?:
If so then you basically just told Visual Studio Code to execute this Python file on your machine. Which probably explains most of your errors. Please note that the Python:
in front of the command means this command comes from the Python extension and not our Torizon extension.
As stated in the example you’re referencing (How to Use CAN on Torizon OS | Toradex Developer Center):
To load this application container in your target, which the Torizon Extension asked for its credentials before (hostname/IP, user, and password, for example), you can just press F5 on your keyboard.
Try to only execute Visual Studio Commands that were made for our extension. Such commands will be pre-pended with a Torizon:
or something similar.
is there an equivalent C example? I am not sure how to combine my existing C project with Python (or do you think I should run this as a separate container and use a docker network to send over the CAN info to my application?)
We only provide this simple Python example as a reference. It’s up to the user to then find the equivalent functionality in whatever programming language they want to use. For C I imagine there’s many examples out there.
Best Regards,
Jeremias
F5 worked. Now I can run this from the debugger.
I tried that the first time and nothing seemed to happen. Perhaps I didn’t wait long enough for all the packages to load…
I have a follow-up questions though…
When I am in my home directory /home/torizon I tried to run the main.py script using python3 and I get the same error. Obviously some things I haven’t managed to understand when it comes to containers/python/CAN etc…
should this not work?
colibri-imx6-10866289:~/CANhandler$ sudo su
Password:
colibri-imx6-10866289:/var/rootdirs/home/torizon/CANhandler# ls
cleanup.sh main.py requirements.txt setup.sh
colibri-imx6-10866289:/var/rootdirs/home/torizon/CANhandler# python3 main.py
Traceback (most recent call last):
File "main.py", line 4, in <module>
import can
ModuleNotFoundError: No module named 'can'
colibri-imx6-10866289:/var/rootdirs/home/torizon/CANhandler#
I’m confused why are you trying to run this outside of a container? The host TorizonCore system wouldn’t even have all the packages needed to run this application. That’s the point of running it in a container so that you can install the packages inside a container and run your app there without having to modify the underlying OS.
As the No module named 'can'
error suggests the application fails because there’s no package on the host OS for this python module. If you take a look at the article again you’ll see that we modify the extrapackages
configuration in the extension to include python3-can
. This is what adds the can
module inside the container, but this is only in the container not outside of it.
Best Regards,
Jeremias
I have no objections to running it from the container if I knew how.
Is there any way to run it besides from the debugger in VScode?
I tried this, but it just seems to hang…
colibri-imx6-10866289:~$ docker run canhandler_arm32v7-debian-python3_bullseye_d
/startptvsd: 2: cd: can't cd to /CANhandler
running CANhandler
^C
exit
^C^C^C
^C^C^C
Are you supposed to be able to open the container in a CLI and run the program from a shell comomand (in order to, for example, also have access to candump and cansend?)
Is there any way to run it besides from the debugger in VScode?
I believe I answered this question on your other post here: Proper method of using docker run command
As a side-note if you’re running the container manually like this you probably want to run the release version of the container rather than the debug version
Best Regards,
Jeremias
Where do you specify that?
I see that tasks.json has the various labels build_debug and build_release and settings.json has “torizon.configuration”: “debug” but I don’t really understand what I am supposed to do to invoke them.
I also see there is a Torizon: C/C++ switch to release configuration… maybe I should click on that…
So much to learn…
I guess there is nothing in the docker command line itself:
docker run --network 'host' --volume /dev:/dev --volume /home/torizon/logs:/home/torizon/logs --volume /run/udev:/run/udev --volume /tmp:/tmp --volume ContainerVolume:/NewVolume --volume /home/torizon/EmeraBlockEnergyBox:/EmeraBlockEnergyBox:rw --device /dev/spidev3.0:/dev/spidev3.0 --device /dev/colibri-i2c:/dev/colibri-i2c --publish :6502/tcp --publish 8080:8080/tcp emerablockenergybox_arm32v7-debian-no-ssh_bullseye_debug_69ac5093-51e4-4f03-8827-ec9f9390dfe3
This is described in our documentation here: IDE Extension | Toradex Developer Center
As the names suggest the debug configuration of the project will run your container in a way that a debug process is attached to it. It will also have extra packages and such related to debugging that you probably don’t want in the final product.
The release configuration is the version of the container that you ideally want to ship in your final product.
The reason I mentioned release vs debug is because you asked:
Is there any way to run it besides from the debugger in VScode?
Therefore you’d build and deploy the release configuration of this project. Then you can either start the release variant of your application container from either VSCode or you can do it yourself manually using the docker run..
command you get from exporting the docker command line in VSCode.
Best Regards,
Jeremias